dom-helpers
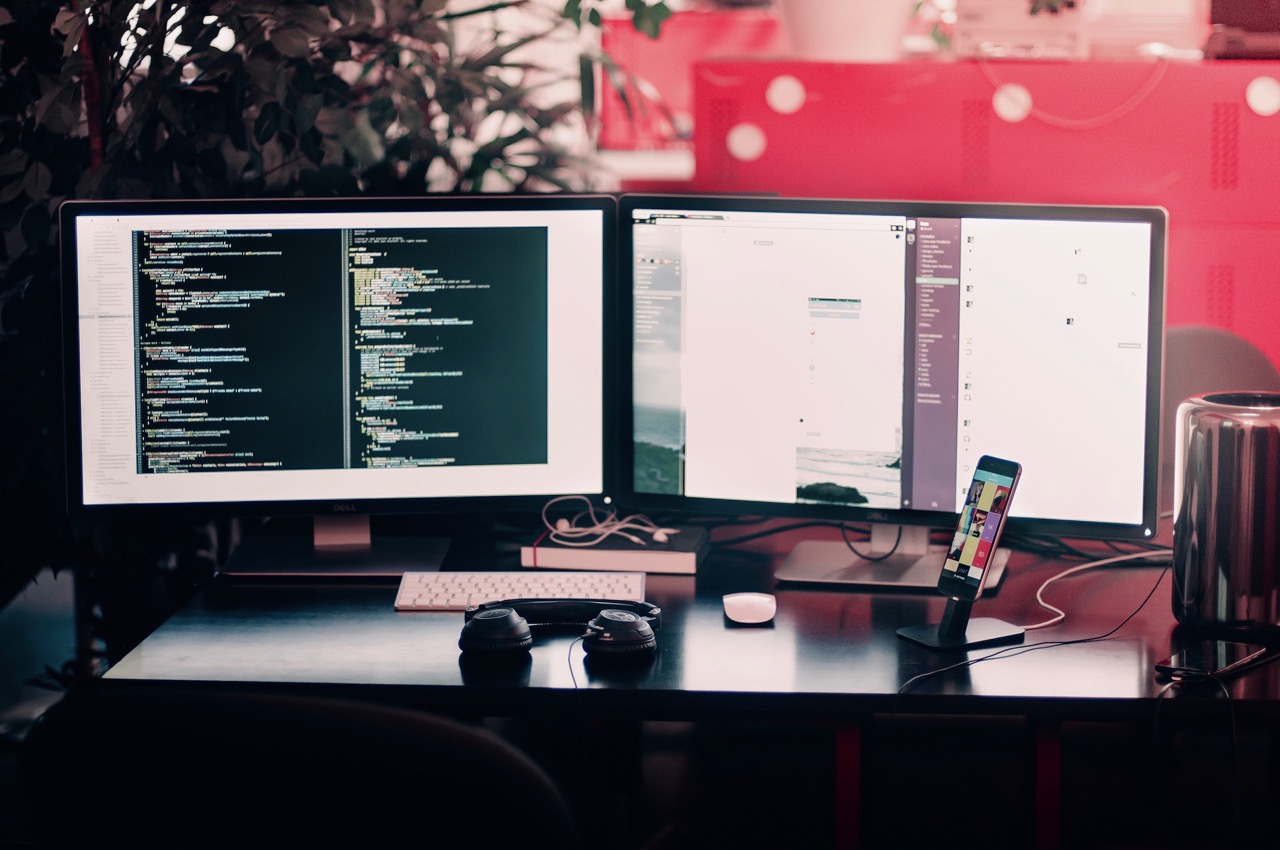
DOM Helpers
Helper functions for faster DOM scripting
Install
yarn add @three11/dom-helpers
or
npm i @three11/dom-helpers
Usage
import {
$,
$$,
hasClass,
addClass,
removeClass,
toggleClass,
insertAfter,
insertBefore,
dispatchEvent,
enableListeners,
getScrollPosition,
isElementVisibleInViewport
} from '@three11/dom-helpers';
or
Import each function separately.
See functions list below:
Functions
$
- queries the DOM and obtains a single element
const button = $('#button');
$$
- queries the DOM and obtains a collection of elements
const buttons = $$('#button');
enableListeners
- enables the custom on
method for attaching of event listeners
enableListeners();
button.on('click', () => {
console.log('clicked a single button');
});
buttons.on('click', () => {
console.log('clicked a button in a collection');
});
isElementVisibleInViewport
- accepts two arguments: DOM element and a boolean flag which states if the element should be partially visible. Returns boolean.
const element = document.getElementById('element');
const isVisible = isElementVisibleInViewport(element, true);
getScrollPosition
- returns the scroll position of the passed DOM Element
const element = document.getElementById('element');
const scrollPosition = getScrollPosition(element);
hasClass
- Returns boolean true if the element has the specified class, false otherwise.addClass
- Adds the specified class to an elementremoveClass
- Removes the specified class from an elementtoggleClass
- Toggles the specified class on an element
const element = document.getElementById('element');
hasClass(element, 'test'); // false
addClass(element, 'test');
removeClass(element, 'test');
/**
* The last argument forces the classname.
* If true the classname will be added,
* if false it will be removed.
* If omitted, the classname will be toggled
*/
toggleClass(element, 'test', true);
insertAfter
- Insert the supplied HTML String after the elementinsertBefore
- Insert the supplied HTML String before the element
const element = document.getElementById('element');
insertAfter(element, '<div>Test</div>');
insertBefore(element, '<div>Test</div>');
dispatchEvent
- Fires a custom (or built-in) event
const element = document.getElementById('element');
// The third argument is event data. Can be omitted
dispatchEvent(element, 'click', { data: true });
License
GNU GENERAL PUBLIC LICENSE Version 3, 29 June 2007